Introduction
Today, users want apps to be fast, easy to use, and responsive. PowerApps is a great tool to build custom apps, and it really can help your business make apps fast. However, the more complex your app becomes, the more chances it may run into performance issues. Slow screens, longer loading times, and unresponsive buttons will annoy users and destroy the good purpose of an app.
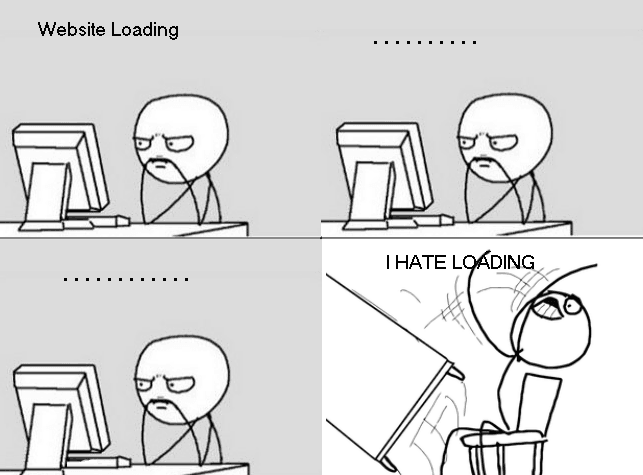
Fortunately, there are good ways to ensure that your PowerApps are always up to the task of providing a great experience for your users. In this article, we shall be showing you some of the best approaches to improve performance in PowerApps, beginning with better data management and ending with the reduction of mess in controls and simplification of app logic. Arrange your apps so they work smoothly, no matter how complicated they become—with these tips.
Let’s dive into the key strategies that will take your PowerApps to the next level!
1. Data Management and Loading Optimization
Utilize Collections and Variables for Data Caching
For faster performance, store frequently accessed data in local collections or variables. This eliminates the need for network calls to a cloud data source, resulting in significantly quicker data retrieval and display.
// Store the categories table in memory for quicker access
ClearCollect(
colCategories,
'categories',
)
Load multiple datasets asynchronously
Imagine you have different independent datasets that you need to load, typically you would make connector call one after the other which might be slow, the solution here is to use the Concurrent function. Concurrent function makes Power Apps to load data immediately using more than one connector call at a time. just keep in mind that it should be used for loading data from the cloud, when you work with data which is already present locally, there is no benefit to use concurrent here.
// Sequential (slower)
ClearCollect( colProducts, Products)
ClearCollect(
colCustomers,
Filter(Customer, Customer.Gender="FEMALE")
)
// Simultaneous (faster)
Concurrent(
// Thread #1
ClearCollect(colProducts, Products),
// Thread #2
ClearCollect(
colCustomers,
Filter(Customer, Customer.Gender="FEMALE")
)
)
Manage Collection Sizes Efficiently
Minimize the number of rows and columns to just what is needed in order to save space in memory. Collections are kept in memory, which may be in short supply. Too much demand on memory can result in application crashes.
Use the ShowColumns
function to select only the required columns and discard unnecessary ones. For Dataverse connections, enable explicit column selection to retrieve only the columns used in your app, further reducing memory usage.
// Retrieving Only desired Columns from the Customers Table
ClearCollect(
colCustomers,
ShowColumns(Customers, "first_name", "last_name", "gender", "phone_number")
)
Eliminate The N+1 Problem
🤔 what is N+1 Problem ?
The N+1 problem is where an application executes N number of unnecessary queries along with one that's actually needed; hence, too many queries are executed on the database. That happens whenever a list of items is retrieved where every item has associated information to be queried separately. Imagine an app being designed to show the gallery of employees; each employee would be associated with a department. First query here fetches the list of employees.
// ITEMS property of the gallery
Employees
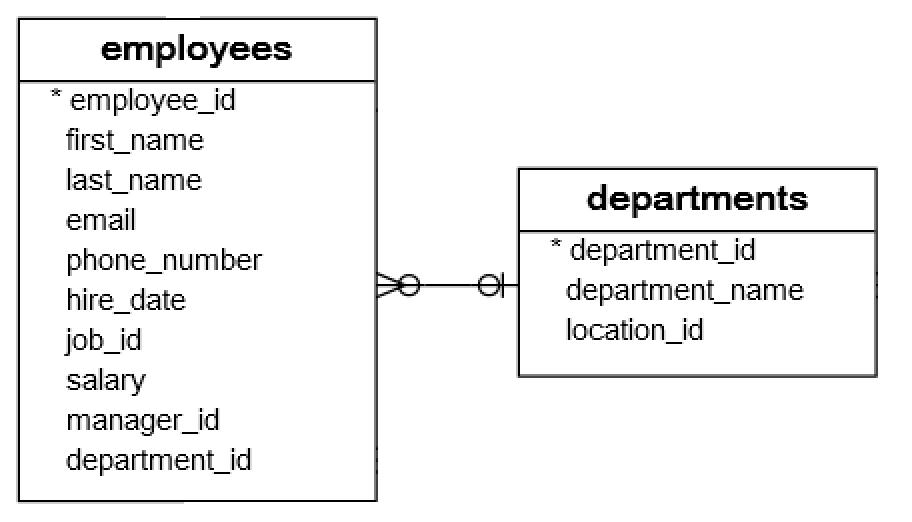
To display the name of the department for each employee, you put a label in the gallery with a lookup function in its text property. The above configuration has as result one query for the first list of employees, plus one more query for each employee's department. If you have 100 employees, you will generate 101 queries, 1 initial and 100 lookups.
// TEXT property of the department name label
LookUp(Departments, ID=ThisItem.department_id, 'department_name')
💡 Optimized Solution for Dataverse
Dataverse can further optimize this and pull associated data in. On the 'retrieve' call for a list of employees, it should return associated department details in one call versus making the call for multiples queries.
// TEXT property of the department name label
ThisItem.Department.'department_name'
🧪 Work around for SharePoint
Unlike Dataverse, SharePoint lists natively do not support complex relational queries-in other words, you cannot completely avoid the N+1 problem. But you can minimize the number of data calls-fetch all the required data before the gallery screen loads. Collect all employee and department data into collections Simultaneously : Now, merge the department names into the employee collection so that you can show all the required data from one source.
// Pre-fetch all employees and departments Simultaneously
Concurrent(
ClearCollect(colDepartments, Departments),
ClearCollect(colEmployees, Employees)
)
// Combine data to include department names
ClearCollect(
colGalleryData,
AddColumns(
colEmployees,
"DepartmentName",
LookUp(colDepartments, ID=ThisItem.DepartmentID, 'Department Name')
)
);
// Use the combined collection for the gallery
colGalleryData
Perform Bulk Updates
Update several records at once within the same datasource table using the “batch patch” approach. "Batch patch" will allow you to make simultaneous updates of the records. The classic method of ForAll and Patch is slower since it does it sequentially.
/**
asume we have a Collection of records
to update, named colUpdateEmployees
*/
// Update records one-by-one (slower)
ForAll(
colUpdateEmployees,
Patch(
Employees,
LookUp(Employees, ID=colUpdateEmployees[@ID]),
{
FullName: colUpdateEmployees[@FullName],
Active: colUpdateEmployees[@Active]
}
)
);
// Bulk update multiple records at once (faster)
Patch(
Employees,
ShowColumns(colUpdateEmployees, "ID", "FullName", "Active")
);
2. Control and UI Optimization
Limit the Number of Controls per Screen
Each added control onto a screen uses more memory when that screen is loaded. This means it should have as little controls as possible on the design of a screen. A screen with fewer controls on it will render faster and will be using less memory. If there are too many controls for a screen, then split their functions across multiple screens.
Use a gallery to display repetitive controls. Each control in a gallery only counts as 1 control no matter how many times it is shown.
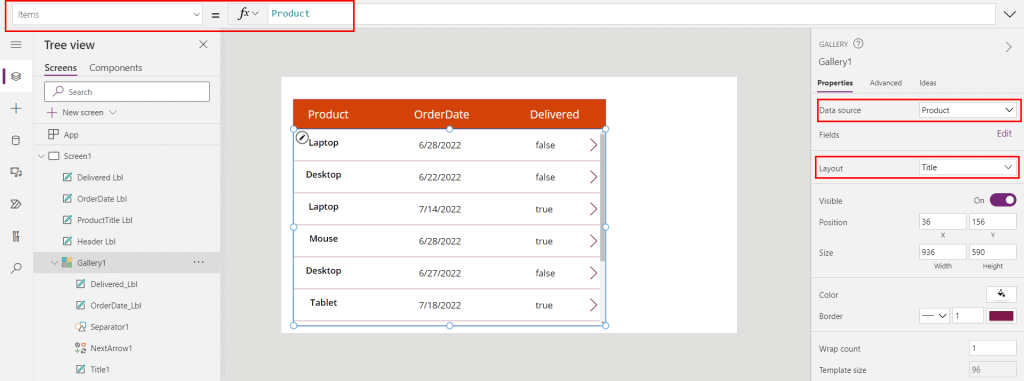
Activate DelayOutput for Text Input Controls
The Text property of a text input is updated after each keystroke. Set the DelayOutput property of the input to true to wait until after the user stops typing. This is useful when building a search bar connected to a gallery. With DelayOutput enabled the app will only make one request to the datasource when typing stops, as opposed to each keystroke.

To enable for a classic text input control, simply select the text input control and find the DelayOutput property:

For a modern text input control, you’ll need to update the Trigger Output property to Delayed. At time of writing the modern text input control is still in preview, so not yet ready for your production apps.

Screen Referencing Best Practice
When creating formulas in Power Apps, it's important to limit references strictly to controls within the same screen. Referencing controls across different screens can lead to performance issues as it forces Power Apps to retain those screens in memory, even when they're not visible on the device. To avoid this, store values from other screens in global variables, and use these variables in your formulas instead.
3. App Logic and Formula Optimization
Keep recently visited screens in memory
This setting allows PowerApps to retain recently visited screens in memory to enhance navigation performance. When enabled, users can switch between screens more quickly since the app doesn’t have to reload them from scratch every time. Just keep in mind this may consume more device memory, which can be an issue for lower-end devices or large applications.
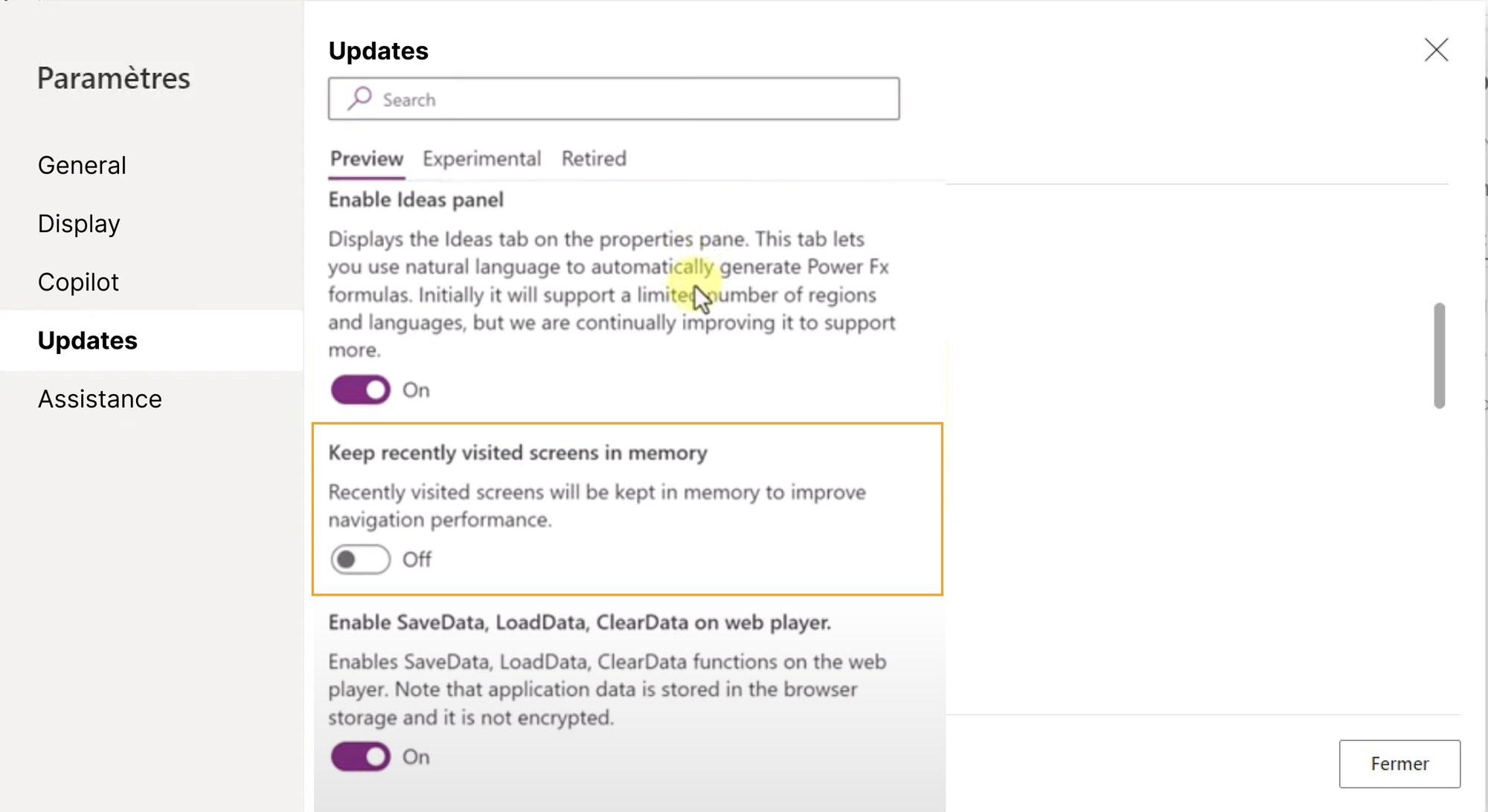
Craft Formulas That Leverage Delegation
Write formulas where possible that can be delegated to the cloud datasource. Delegation means that operations such as filtering, lookups, and searches are processed in the cloud - for example, within SharePoint or Dataverse - and instead on the user's device. This has a double advantage of speeding up data operations on much stronger processors in the cloud but also reduces the amount of data to be downloaded onto the user's device because the filtering is done at the source.
here are the list of delegable functions for :
And remember checking warnings since a warning will appear in the app checker when a function cannot be delegated.
Bonus: For cases where Power Apps formulas don’t support delegation, Dataverse views provide an alternative, as they are not subject to delegation rules. Use Dataverse views to apply filter criteria that would otherwise be non-delegable in Power Apps.
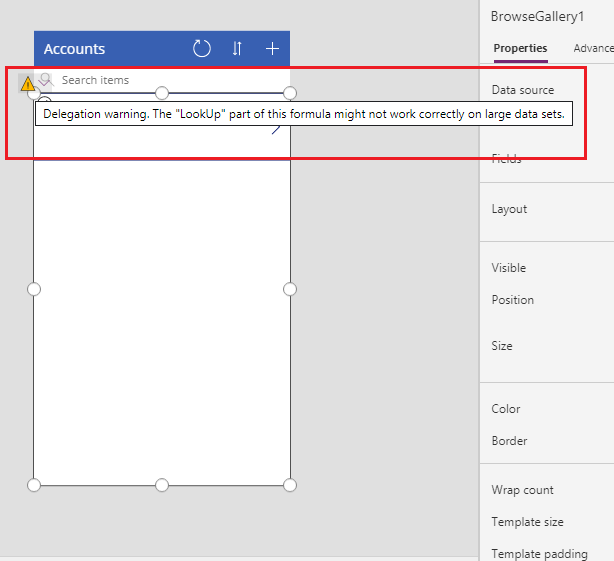
Conclusion
In summary, any optimization for any application can only be done by improving the handling of data to be more efficient, simplifying the UI design, and making sure presentation logic is clear. This will result in applications that run smoother and faster due to avoiding extra processing of data, reduction in memory usage, and hence making them responsive. A better user experience comes with a simpler interface, fewer elements, and no unnecessary links between components.
There is no doubt that performance optimization of Power Apps is achievable through adherence to a set of best practices, including data management optimization, UI design refinement, and improvement of app logic. These apps need to remain responsive as their complexity increases to keep the user experience very good.
A good app really raises the happiness level of users and makes your solution work even better. Now, apply these strategies and make real success take off with Power Apps!